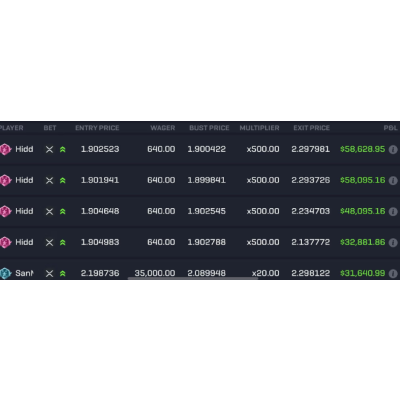
In this guide, you’ll learn how to build a Crypto trading Bot for Binance Futures Testnet using Python. The bot will be able to connect to the Binance exchange and execute orders based on your strategy.
The trading strategy is very simple, the bot will trade Bitcoin (BTCUSDT) on Binance Testnet and monitor the price continuously. If the price of Bitcoin goes up 2% it sells a portion of your Bitcoin. Now if the price of Bitcoin goes down 2% the bot will buy.
Basically the bot will automate the process of buying or selling Bitcoin on the Binance Futures Testnet based on a 2% price change. For this guide, I’m using the Testnet also known as Mock Trading which allows users to practice trading without losing real money.
With no real money involved, you can experiment, make mistakes, and learn without the fear of losing money.
First, you will need to install some Python libraries. Open a terminal and run the following command to install the required libraries:
pip install python-binance pandas numpy
Now, let’s move to the next step.
To find the API keys on the Binance Futures Testnet, go to https://testnet.binancefuture.com/en/futures/BTCUSDT and create an account if you don’t already have one.
Then look for “API Key, " copy both API Key and API Secret, and save them. You will need these keys in the next step to connect to the Binance exchange.
In this step, I will create a new file by using nano text editor.
nano binancebot.py
Add the following code:
import os
import time
import logging
from binance.client import Client
from binance.enums import ORDER_TYPE_MARKET, SIDE_BUY, SIDE_SELL
# Set up logging
logging.basicConfig(level=logging.INFO, format='%(asctime)s - %(levelname)s - %(message)s')
# Directly set your Testnet API key and secret here (for debugging purposes)
api_key = 'your_testnet_api_key' # Replace with your actual Testnet API key
api_secret = 'your_testnet_api_secret' # Replace with your actual Testnet API secret
# Initialize Binance Client for Testnet
client = Client(api_key, api_secret, testnet=True)
# Check the current position mode (One-way or Hedge mode)
position_mode_info = client.futures_get_position_mode()
position_mode = position_mode_info['dualSidePosition']
logging.info(f"Account Position Mode: {'Hedge Mode' if position_mode else 'One-way Mode'}")
# Initialize the initial price to None
initial_price = None
def get_current_price(symbol):
"""Fetch the current price of the given symbol."""
ticker = client.futures_symbol_ticker(symbol=symbol)
return float(ticker['price'])
def place_order(symbol, side, quantity, position_mode, position_side=None):
"""Place a market order on Binance Futures Testnet."""
try:
if position_mode:
# Hedge Mode: Use positionSide parameter
order = client.futures_create_order(
symbol=symbol,
side=side,
type=ORDER_TYPE_MARKET,
quantity=quantity,
positionSide=position_side # SHORT or LONG
)
else:
# One-way Mode: Do not use positionSide parameter
order = client.futures_create_order(
symbol=symbol,
side=side,
type=ORDER_TYPE_MARKET,
quantity=quantity
)
logging.info(f"Order placed: {order}")
except Exception as e:
logging.error(f"An error occurred: {e}")
# Define the trading parameters
symbol = 'BTCUSDT'
quantity = 0.005 # Adjust this value to change the order size
while True:
try:
current_price = get_current_price(symbol)
if initial_price is None:
initial_price = current_price
price_change = (current_price - initial_price) / initial_price * 100
if price_change >= 2:
# Sell if price goes up by 2%
place_order(symbol, SIDE_SELL, quantity, position_mode, 'SHORT' if position_mode else None)
initial_price = current_price # Reset initial price after trade
elif price_change <= -2:
# Buy if price goes down by 2%
place_order(symbol, SIDE_BUY, quantity, position_mode, 'LONG' if position_mode else None)
initial_price = current_price # Reset initial price after trade
logging.info(f"Current price: {current_price}, Price change: {price_change}%")
time.sleep(60)
except Exception as e:
logging.error(f"An error occurred: {e}")
time.sleep(60)
Replace ‘your_testnet_api_key’ and ‘your_testnet_api_secret’ with your Testnet API key and secret from Binance.
symbol = ‘BTCUSDT’: This line sets the trading pair to be used in the trading strategy. In this case, it is ‘BTCUSDT’.
quantity = 0.005: This line sets the quantity of Bitcoin to be traded in each order. Here, it is set to 0.005 BTC.
You can adjust this value to change the size of each trade. For example, if you want to trade a larger or smaller amount of Bitcoin, you can change 0.005 to your desired quantity.
Open a terminal and go to the directory where “binancebot.py” is saved and type the following command:
python3 binancebot.py
The bot will now start monitoring the BTCUSDT price and make trades whenever the price changes by +2% or -2%.
To make a quick summary the bot fetches the current price of BTCUSDT and compares it to the initial price.
If the price increases by 2%, it places a sell order for 0.005 BTC. If the price decreases by 2% or more, it places a buy order for 0.005 BTC. You can also see the percentage change for the price in real time.
Now if a buy/sell order is placed, you’ll get a message on your terminal saying “Order placed”.
At this point, you can go to Binance and check the bot activity by clicking “Trade History” to see all the trades that have been executed.
In this tutorial, you’ve discovered how to create a cryptocurrency trading bot using Python. You can expand the bot by adding more strategies, implementing risk management, and exploring other trading pairs.