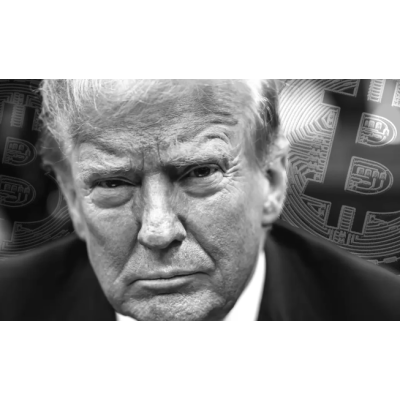
But, here’s a heads-up: trading fees can be a real game-changer. When I was testing this script, I found that the fees from each trade started eating into my profits, and before I knew it, I was actually losing money instead of making it. It was a bit of an “aha” moment for me about just how crucial it is to factor in those fees when you’re planning your trading strategy.
When I was testing this script, I found that the fees from each trade started eating into my profits, and before I knew it, I was actually losing money instead of making it.
That “aha” moment really hits you like a splash of cold water, doesn’t it? You’ve set up your trading bot, all excited about the wonders of automation. It’s churning away, buying low and selling at that sweet 3% higher price point the linear regression algorithm has determined. You’re practically rubbing your hands together, waiting for the profits to roll in.
But then, reality strikes. Coinbase, with its trading fees, steps in and takes a hefty percent off your transactions. Suddenly, your 3% gain doesn’t just shrink; it vanishes into thin air, leaving you with less than you started. It’s like running a lap and realizing you’re further from where you began. It’s in these moments you truly understand the critical importance of accounting for trading fees in your strategy. It’s not just about the gains; it’s about what’s left after the fees have had their bite.
So, as you play around with this starter script, just keep in mind it’s really just the first step. It’s meant to help you get the hang of connecting your algo-trading ideas to Coinbase, but don’t stop there. The crypto market is super dynamic, and you’ll need to keep tweaking and adjusting your strategy, especially considering those pesky trading fees, to stay ahead of the game.
Diving into the world of automated trading has been quite the adventure, full of ups, downs, and a few facepalm moments. One of the quirky things I had to figure out the hard way was dealing with the Coinbase API and its not-so-friendly attitude towards decimal points in orders. Picture this: you’ve got your trading bot all set up, ready to make some slick trades, and then bam, the API throws a tantrum because your order amounts have decimals. Talk about being finicky, right?
So, I had to get a bit creative and start rounding down my balances to whole numbers before even thinking about making a purchase. Who knew a little rounding could be the peacekeeper between my bot and the API?
But wait, there’s more. The API also seems to get a bit overwhelmed if your buy or sell orders are for more than what’s available at that price point. It’s like trying to buy ten apples when there are only five on the shelf — it’s just not happening. To work around this, I had to implement loops in the script, constantly tweaking the amount in the orders until the API finally gave the green light. It was a bit of trial and error, but hey, that’s all part of the fun in getting this stuff to work.
So, if you’re venturing into setting up your own trading bot, keep these little nuggets in mind. A bit of rounding here and some persistent looping there could be the difference between a smooth-sailing trading bot and one that’s constantly hitting a wall. Happy trading, and may the odds be ever in your favor (or at least may the API be a little less grumpy)!
First, you’ll need to create your API keys from settings.
pip install coinbase-advancedtrade-python
pip3 install coinbase-advanced-py
pip install coinbase
pip install numpy
pip install pandas
pip install ccxt
This script is pretty much a little digital trader that lives to execute trades on your behalf, based on some smart logic and market predictions. It’s like having a mini broker inside your computer, except this one doesn’t sleep, eat, or take coffee breaks.
import time
from coinbase.wallet.client import Client
from coinbase_advanced_trader.config import set_api_credentials
from coinbase_advanced_trader.strategies.market_order_strategies import fiat_market_sell
from coinbase_advanced_trader.strategies.market_order_strategies import fiat_market_buy
import numpy as np
import pandas as pd
from datetime import datetime
from getpass import getpass # Use getpass for secure input
from ccxt import coinbasepro
from decimal import Decimal
import math
# Set your Coinbase API key and secret
API_KEY = "YOUR COINBASE API KEY"
API_SECRET = "YOUR COINBASE SECRET API KEY"
# Set the API credentials once
set_api_credentials(API_KEY, API_SECRET)
# Fetch historical data and make predictions
def fetch_data_and_predict():
data_prep = []
exchange = coinbasepro() # Renamed variable to avoid conflict
for trade in exchange.fetch_trades('ADA/EUR', limit=1000): # Fetching the latest 1000 trades
time_of_trade = trade['datetime']
trade_price = trade['price']
convert_time_of_trade_to_datetime = datetime.strptime(time_of_trade, "%Y-%m-%dT%H:%M:%S.%fZ")
data_prep.append((convert_time_of_trade_to_datetime, trade_price))
dfa = pd.DataFrame(data_prep, columns=['date', 'Price'])
dfa['date'] = pd.to_datetime(dfa['date']).astype(int) / 10**9 # Convert datetime to Unix timestamp
# Perform linear regression on min and max prices by hour
dfa['hour'] = dfa['date'].apply(lambda x: datetime.fromtimestamp(x).hour)
min_prices = dfa.groupby('hour')['Price'].min().reset_index()
max_prices = dfa.groupby('hour')['Price'].max().reset_index()
min_slope, min_intercept = np.polyfit(min_prices['hour'], min_prices['Price'], 1)
max_slope, max_intercept = np.polyfit(max_prices['hour'], max_prices['Price'], 1)
# Predict next hour's min and max prices
next_hour = max(min_prices['hour'].max(), max_prices['hour'].max()) + 1
predicted_min_price = min_slope * next_hour + min_intercept
predicted_max_price = max_slope * next_hour + max_intercept
# Calculate sell scenario line (top 20% between predicted min and max prices)
top_20_percent_threshold = (predicted_max_price - predicted_min_price) * 0.8
sell_scenario_line = predicted_min_price + top_20_percent_threshold
return predicted_min_price, predicted_max_price, sell_scenario_line
def sell_ada_for_euros(ada_amount):
int(ada_amount)
while ada_amount > 3:
try:
# Define the trading parameters
product_id = "ADA-EUR" # Replace with the desired trading pair
ada_size = int(ada_amount) # Specify the amount of ADA you want to sell
print(ada_size)
# Perform a market sell
market_sell_order = fiat_market_sell(product_id, ada_size)
# Print the order details
print(f"Successfully sold {market_sell_order['filled_size']} ADA at the current market price.")
print(f"Order ID: {market_sell_order['order_id']}")
break # Exit the loop if purchase is successful
except Exception as e:
print(f"Error: {e}")
ada_amount -= 1 # Reduce the euro amount by 1 and try again
def buy_ada_with_euros(euro_amount):
int(euro_amount)
while euro_amount > 3: # Ensure there's at least 1 euro to try buying ADA
try:
# Define the trading parameters
product_id = "ADA-EUR" # Replace with the desired trading pair
ada_size = euro_amount # Attempt to use the current euro amount
# Perform a market buy
market_buy_order = fiat_market_buy(product_id, ada_size)
# Print the order details
print(f"Successfully bought ADA with {euro_amount} euros at the current market price.")
print(f"Order ID: {market_buy_order['order_id']}")
break # Exit the loop if purchase is successful
except Exception as e:
print(f"Error with {euro_amount} euros: {e}")
euro_amount -= 1 # Reduce the euro amount by 1 and try again
def execute_trades(client, predicted_min_price, predicted_max_price, sell_scenario_line):
# Fetch the current price of ADA/EUR
current_price = float(client.get_spot_price(currency_pair='ADA-EUR')['amount'])
# Fetch account balances
eur_account = client.get_account('EUR')
ada_account = client.get_account('ADA')
# Check and print balances
eur_balance = float(eur_account.balance.amount) if eur_account else 0
ada_balance = float(ada_account.balance.amount) if ada_account else 0
print(f"EUR Balance: {eur_balance}, ADA Balance: {ada_balance}")
# Proceed with trading logic only if accounts are found
if eur_account and ada_account:
if current_price <= predicted_min_price and eur_balance > 3:
eur_balance = math.floor(eur_balance * 100) / 100.0
print(type(eur_balance))
print("Buy order would be placed using entire EUR balance:", eur_balance)
# Place buy order
try:
# Call the function to buy ADA with Euros
Decimal(eur_balance)
buy_ada_with_euros(eur_balance)
print("Buy order placed:", eur_balance)
except Exception as e:
print(f"An error occurred: {e}")
elif current_price >= sell_scenario_line and ada_balance > 3:
ada_balance = math.floor(ada_balance * 100) / 100.0
print(type(ada_balance))
print("Sell order would be placed using entire ADA balance:", ada_balance)
# Place sell order
Decimal(ada_balance)
sell_ada_for_euros(ada_balance)
print("Sell order placed:", ada_balance)
else:
print("Hold. Current price is:", current_price)
print(predicted_min_price, predicted_max_price, sell_scenario_line)
# Hold logic here
else:
print("Missing EUR or ADA account. Unable to execute trades.")
# Set your Coinbase API credentials
api_key = 'YOUR COINBASE API KEY'
api_secret = 'YOUR COINBASE SECRET API KEY'
client = Client(api_key, api_secret)
def job():
# Your existing code to fetch predictions and execute trades
predicted_min_price, predicted_max_price, sell_scenario_line = fetch_data_and_predict()
execute_trades(client, predicted_min_price, predicted_max_price, sell_scenario_line)
# Keep the script running
while True:
time.sleep(60)
job()
Here’s a rundown of what’s happening under the hood:
It’s important to note that while this script is a great starting point for anyone looking to get their feet wet in automated trading on Coinbase, it’s just the beginning. The world of crypto trading is vast and ever-changing, so continuous learning, tweaking, and adapting are part of the game. Happy trading, and may your digital broker bring you plenty of successful trades!