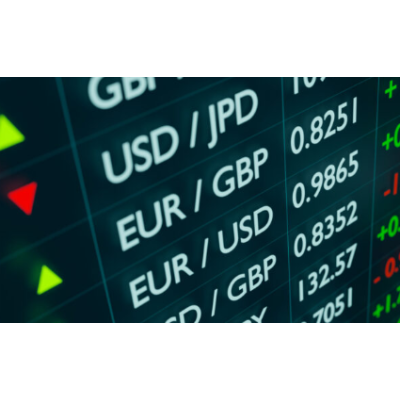
With just a few lines of Python code, you can unleash a powerful trading algorithm that transforms complex market analysis into actionable trading strategies.
This isn’t science fiction — it’s the reality of algorithmic trading, where technology meets finance in a symphony of data-driven decision-making.
In this blog, we’ll show you how you can deploy an advanced trading algorithm with minimal coding effort, leaving it to run autonomously.
Whether you’re catching up on sleep, exploring new hobbies, or simply enjoying time with loved ones, your personal trading assistant works tirelessly, scanning the market to buy, sell, or hold based on meticulously calculated indicators.
By the end of this guide, you’ll have unlocked the potential to engage with the cryptocurrency market like never before, all with just a few keystrokes and the power of algorithmic trading at your fingertips. Welcome to the future of trading, where efficiency, precision, and autonomy are just the beginning.
The algorithm operates by first collecting a large dataset of historical trade data, focusing on price fluctuations over time. It then applies linear regression analysis to this data to identify the minimum and maximum price trends for each hour, effectively mapping out the price landscape.
With these trends established, the algorithm forecasts the upcoming minimum and maximum prices for the next hour. Utilizing these predictions, it calculates a sell threshold within the top 20% range between the predicted minimum and maximum prices, establishing a refined point at which selling is deemed optimal.
In real-time operation, the algorithm continuously compares the current market price against these calculated thresholds to make informed decisions: if the current price falls below the predicted minimum, it triggers a buy order; if the price reaches or surpasses the sell threshold within the top 20% range, it initiates a sell order; otherwise, it recommends holding if the price is between these two points but doesn’t meet the specific conditions for buying or selling.
This systematic approach allows for dynamic and responsive trading strategies based on predictive analytics and real-time market data.
2. Buy Scenario (Middle Graph):
3. Hold Scenario (Right Graph):
These visualizations help illustrate how the trading algorithm could use the adjusted sell threshold, alongside the predicted price trends, to make informed decisions on when to buy, sell, or hold, based on the current market price’s position relative to these trends. You would also need to factor in transaction costs.
import ccxt
import numpy as np
import pandas as pd
from datetime import datetime
import cbpro
import passwords # Ensure you have a passwords.py file with api_key, api_secret, and api_passphrase
# Fetch historical data and make predictions
def fetch_data_and_predict():
data_prep = []
coinbasepro = ccxt.coinbasepro()
for trade in coinbasepro.fetch_trades('ADA/EUR', limit=1000): # Fetching the latest 1000 trades
time_of_trade = trade['datetime']
trade_price = trade['price']
convert_time_of_trade_to_datetime = datetime.strptime(time_of_trade, "%Y-%m-%dT%H:%M:%S.%fZ")
data_prep.append((convert_time_of_trade_to_datetime, trade_price))
dfa = pd.DataFrame(data_prep, columns=['date', 'Price'])
dfa['date'] = pd.to_datetime(dfa['date']).astype(int) / 10**9 # Convert datetime to Unix timestamp
# Perform linear regression on min and max prices by hour
dfa['hour'] = dfa['date'].apply(lambda x: datetime.fromtimestamp(x).hour)
min_prices = dfa.groupby('hour')['Price'].min().reset_index()
max_prices = dfa.groupby('hour')['Price'].max().reset_index()
min_slope, min_intercept = np.polyfit(min_prices['hour'], min_prices['Price'], 1)
max_slope, max_intercept = np.polyfit(max_prices['hour'], max_prices['Price'], 1)
# Predict next hour's min and max prices
next_hour = max(min_prices['hour'].max(), max_prices['hour'].max()) + 1
predicted_min_price = min_slope * next_hour + min_intercept
predicted_max_price = max_slope * next_hour + max_intercept
# Calculate sell scenario line (top 20% between predicted min and max prices)
top_20_percent_threshold = (predicted_max_price - predicted_min_price) * 0.8
sell_scenario_line = predicted_min_price + top_20_percent_threshold
return predicted_min_price, predicted_max_price, sell_scenario_line
# Authenticate with the Coinbasepro API
def authenticate():
return cbpro.AuthenticatedClient(passwords.api_key, passwords.api_secret, passwords.api_passphrase)
# Execute trades based on predictions and current price
def execute_trades(client, predicted_min_price, predicted_max_price, sell_scenario_line):
current_price = float(client.get_product_ticker(product_id='ADA/EUR')['price'])
# Fetch account balances
accounts = client.get_accounts()
eur_account = next(acc for acc in accounts if acc['currency'] == 'EUR')
ada_account = next(acc for acc in accounts if acc['currency'] == 'ADA')
eur_balance = float(eur_account['available'])
ada_balance = float(ada_account['available'])
if current_price <= predicted_min_price and eur_balance > 0:
# Execute a buy order using the entire EUR balance
buy_order = client.place_market_order(product_id='ADA/EUR', side='buy', funds=str(eur_balance))
print("Buy order placed using entire EUR balance:", eur_balance, "| Order details:", buy_order)
elif current_price >= sell_scenario_line and ada_balance > 0:
# Execute a sell order using the entire ADA balance
sell_order = client.place_market_order(product_id='ADA/EUR', side='sell', size=str(ada_balance))
print("Sell order placed using entire ADA balance:", ada_balance, "| Order details:", sell_order)
else:
# Hold if conditions for buying or selling are not met
print("Hold. Current price is:", current_price)
def main():
client = authenticate()
predicted_min_price, predicted_max_price, sell_scenario_line = fetch_data_and_predict()
execute_trades(client, predicted_min_price, predicted_max_price, sell_scenario_line)
if __name__ == "__main__":
main()
The provided code snippet outlines the structure of a cryptocurrency trading bot designed to operate on the Coinbase Pro exchange. This trading bot leverages historical trade data and predictive analytics to autonomously make buy, sell, or hold decisions based on real-time market conditions. Here’s a breakdown of what the code is doing:
1. Data Collection: The bot starts by fetching historical trading data for a specified cryptocurrency pair (e.g., ADA/EUR) from Coinbase Pro. It collects details of recent trades, including the time and price of each trade, and organizes this information into a structured format suitable for analysis.
2. Data Preparation and Analysis: The collected trade data is converted into a pandas DataFrame, a popular data manipulation library in Python, facilitating easier data analysis. The code then segments the data by hour and calculates the minimum and maximum prices for each hour, setting the stage for trend analysis.
3. Trend Prediction: Using linear regression, a basic yet powerful statistical method, the code predicts future price trends based on the hourly minimum and maximum prices. This involves calculating the slope and intercept for both the minimum and maximum price trends, which are then used to estimate the prices for the next hour.
4. Decision Thresholds: The bot calculates a sell threshold within the top 20% range between the predicted minimum and maximum prices. This threshold is critical for identifying optimal selling points, aiming to maximize profits by suggesting sales before potential downturns.
5. Trading Decisions: With the sell threshold established, the bot evaluates the current market price against this threshold and the predicted minimum price. If the current price is below the predicted minimum, indicating a potential low, the bot places a buy order. Conversely, if the current price meets or exceeds the sell threshold, suggesting a high, the bot executes a sell order. In cases where the price falls between these parameters without a clear signal, the bot opts to hold, awaiting more favorable conditions.
6. Authentication and Execution: The code includes functions to authenticate with the Coinbase Pro API using security credentials (API key, secret, and passphrase). Once authenticated, the bot can execute trades based on its predictions and the current market price, directly interacting with the exchange to place buy or sell orders as needed.
In essence, this trading bot automates the process of analyzing market trends, predicting future movements, and making informed trading decisions, allowing users to potentially capitalize on market opportunities with minimal manual intervention.