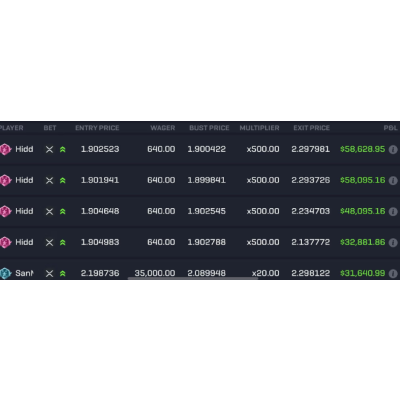
In the dynamic world of options trading, the ability to automate strategies can be a game-changer. Trading bots, when designed effectively, can execute trades with precision, speed, and consistency. In this guide, we’ll delve deep into the development of a trading bot for Long Put and Short Put strategies. By the end, you’ll have a clear roadmap to harness the power of automation for these popular trading strategies.
In the unpredictable world of trading, a Long Put offers a protective shield against potential declines in an underlying asset’s price. By automating this strategy, traders can capitalize on bearish market movements without the need for constant monitoring. Automation ensures that the strategy is executed at the right moment, especially in volatile markets where prices can change rapidly.
def long_put_trade(asset_price, strike_price, expiration_date):
if asset_price < strike_price:
return "Buy Put Option"
else:
return "Hold"
Short Put:
The Short Put strategy is an excellent tool for traders who have a bullish to neutral outlook on an asset. By selling a put option, traders can collect premiums, which can be a consistent income source. Automating this strategy is beneficial for managing multiple positions simultaneously and ensuring that the trader capitalizes on optimal premium collection opportunities without being glued to the screen.
def short_put_trade(asset_price, strike_price, premium):
if asset_price > strike_price:
return f"Collect {premium} premium"
else:
return "Prepare to buy the asset"
A well-structured development environment is the backbone of any successful software project. For a trading bot, it’s even more critical as it ensures efficient coding, seamless testing, and smooth deployment. A good environment will also provide tools for debugging, version control, and collaboration, making the development process more streamlined and error-free.
pandas
for data manipulation, numpy
for numerical operations, and yfinance
for fetching financial data are essential for our bot. These libraries provide pre-built functions and methods that can significantly speed up the development process.# Setting up a virtual environment
python -m venv trading_bot_env
source trading_bot_env/bin/activate
# Installing necessary libraries
pip install pandas numpy yfinance
# Initializing a Git repository
git init
flake8
or pylint
to maintain code quality. Continuous Integration (CI) tools can also be set up to automate testing and deployment processes.import yfinance as yf
# Fetching historical data for Apple from 2020 to 2023
data = yf.download("AAPL", start="2020-01-01", end="2023-01-01")
pandas
library is particularly useful for manipulating and analyzing financial data.import pandas as pd
# Calculating a 50-day moving average
data['50_MA'] = data['Close'].rolling(window=50).mean()
# Identifying potential buy signals when price crosses above the 50-day MA
data['Buy_Signal'] = data['Close'] > data['50_MA']
from sqlalchemy import create_engine
# Setting up a connection to a PostgreSQL database
engine = create_engine('postgresql://username:password@localhost:5432/mydatabase')
# Storing the data in the database
data.to_sql('apple_data', engine, if_exists='replace')
matplotlib
and seaborn
allow for the creation of charts and graphs, aiding in strategy refinement.import matplotlib.pyplot as plt
# Plotting Apple's closing price and 50-day moving average
plt.figure(figsize=(12,6))
plt.plot(data['Close'], label='Close Price')
plt.plot(data['50_MA'], label='50-Day MA', linestyle='--')
plt.legend()
plt.title('Apple Stock Analysis')
plt.show()
def long_put_logic(asset_price, moving_average):
if asset_price < moving_average:
return "Execute Long Put"
return "Hold"
def short_put_logic(volatility, threshold):
if volatility > threshold:
return "Execute Short Put"
return "Hold"
# Example: Using RSI for decision-making
def rsi_logic(rsi_value):
if rsi_value > 70:
return "Overbought - Potential Short Opportunity"
elif rsi_value < 30:
return "Oversold - Potential Long Opportunity"
return "Neutral"
try:
# Attempt to fetch data or execute trade
except Exception as e:
print(f"Error encountered: {e}")
# Implement fallback logic or notification system
start_date = "2015-01-01"
end_date = "2020-01-01"
for date, row in historical_data.iterrows():
action = trading_algorithm(row)
simulate_trade(action, row)
def check_exit_conditions(current_price, entry_price, stop_loss, take_profit):
if current_price <= entry_price - stop_loss:
return "Sell - Stop Loss Triggered"
elif current_price >= entry_price + take_profit:
return "Sell - Take Profit Triggered"
return "Hold"
# Example: Using a broker's API to place a trade
broker_api.place_order(asset="AAPL", action="BUY", quantity=10)
if current_drawdown > max_allowed_drawdown:
send_alert("Drawdown Limit Exceeded!")
import yfinance as yf
import pandas as pd
from sqlalchemy import create_engine
import matplotlib.pyplot as plt
# Data Acquisition
def fetch_data(ticker, start_date, end_date):
return yf.download(ticker, start=start_date, end=end_date)
# Long Put Logic
def long_put_logic(asset_price, moving_average):
if asset_price < moving_average:
return "Execute Long Put"
return "Hold"
# Short Put Logic
def short_put_logic(volatility, threshold):
if volatility > threshold:
return "Execute Short Put"
return "Hold"
# RSI Logic (Placeholder for demonstration)
def rsi_logic(rsi_value):
if rsi_value > 70:
return "Overbought - Potential Short Opportunity"
elif rsi_value < 30:
return "Oversold - Potential Long Opportunity"
return "Neutral"
# Exit Conditions
def check_exit_conditions(current_price, entry_price, stop_loss, take_profit):
if current_price <= entry_price - stop_loss:
return "Sell - Stop Loss Triggered"
elif current_price >= entry_price + take_profit:
return "Sell - Take Profit Triggered"
return "Hold"
# Placeholder Broker API (For demonstration purposes)
class BrokerAPI:
def place_order(self, asset, action, quantity):
print(f"Placing order: {action} {quantity} of {asset}")
# Main Function
def main():
# Fetching data
data = fetch_data("AAPL", "2020-01-01", "2023-01-01")
# Calculating a 50-day moving average
data['50_MA'] = data['Close'].rolling(window=50).mean()
# Simulating trades (simplified for demonstration)
for date, row in data.iterrows():
action = long_put_logic(row['Close'], row['50_MA'])
if action == "Execute Long Put":
print(f"{date}: {action}")
# Visualization
plt.figure(figsize=(12,6))
plt.plot(data['Close'], label='Close Price')
plt.plot(data['50_MA'], label='50-Day MA', linestyle='--')
plt.legend()
plt.title('Apple Stock Analysis')
plt.show()
# Placeholder for Volatility Calculation (For demonstration purposes)
def calculate_volatility(data):
return data['Close'].rolling(window=50).std()
# Risk Management
def manage_risk(current_price, entry_price, stop_loss, take_profit):
action = check_exit_conditions(current_price, entry_price, stop_loss, take_profit)
if action != "Hold":
print(action)
return action
# Deployment & Monitoring
def deploy_bot():
# Placeholder for a cloud deployment setup
print("Deploying bot to cloud...")
# Placeholder for monitoring setup
print("Setting up monitoring tools...")
# Main Function
def main():
# Fetching data
data = fetch_data("AAPL", "2020-01-01", "2023-01-01")
# Calculating a 50-day moving average
data['50_MA'] = data['Close'].rolling(window=50).mean()
# Calculating volatility (simplified)
data['Volatility'] = calculate_volatility(data)
# Simulating trades (simplified for demonstration)
broker = BrokerAPI()
for date, row in data.iterrows():
long_put_action = long_put_logic(row['Close'], row['50_MA'])
short_put_action = short_put_logic(row['Volatility'], 2) # Assuming a threshold of 2 for simplicity
if long_put_action == "Execute Long Put":
print(f"{date}: {long_put_action}")
broker.place_order("AAPL", "BUY", 1) # Assuming buying 1 option for simplicity
elif short_put_action == "Execute Short Put":
print(f"{date}: {short_put_action}")
broker.place_order("AAPL", "SELL", 1) # Assuming selling 1 option for simplicity
# Risk management check
manage_risk(row['Close'], row['50_MA'], 5, 10) # Assuming stop loss of $5 and take profit of $10 for simplicity
# Visualization
plt.figure(figsize=(12,6))
plt.plot(data['Close'], label='Close Price')
plt.plot(data['50_MA'], label='50-Day MA', linestyle='--')
plt.legend()
plt.title('Apple Stock Analysis')
plt.show()
# Deploying and monitoring the bot
deploy_bot()
# Execute the main function
if __name__ == "__main__":
main()