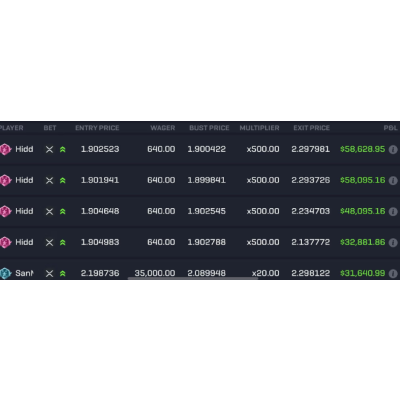
Automation has become a game-changer. Trading bots, powered by algorithms, can execute trades at lightning speed, capitalizing on market inefficiencies. One of the most intriguing strategies is the “Risk On Risk Off” (RORO) approach. This article will guide you through creating a RORO trading bot, ensuring you harness the power of automation while managing risks effectively.
Before diving into code, it’s crucial to grasp the RORO concept. At its core, RORO is about adjusting your trading strategy based on market volatility. In ‘Risk On’ scenarios, traders are bullish and invest in higher-risk assets. Conversely, in ‘Risk Off’ situations, they’re bearish, opting for safer assets.
The language you choose should be compatible with the trading platform’s API and be efficient for data processing. Python, with its extensive libraries like Pandas and NumPy, is a popular choice.
import pandas as pd
import numpy as np
The heart of any trading bot lies in its ability to interact seamlessly with a trading platform. This integration is vital because it allows the bot to fetch real-time market data, execute trades, and manage portfolios. Without this bridge, your bot would be like a car without fuel.
Before you even start coding, you need to decide on which trading platform to integrate with. Factors to consider include:
Most modern trading platforms offer API access. This typically involves:
API_KEY = 'YOUR_API_KEY'
API_SECRET = 'YOUR_API_SECRET'
Once you have your API keys, it’s time to establish a connection. Using Python, this often involves importing the platform’s specific library and initializing a connection.
For MetaTrader5
import MetaTrader5 as mt5
if not mt5.initialize():
print("Initialization failed")
mt5.shutdown()
For Binance
from binance.client import Client
client = Client(API_KEY, API_SECRET)
With the connection established, you can now fetch market data. This is crucial for your bot to make informed decisions.
Fetching Market Data:
With the connection established, you can now fetch market data. This is crucial for your bot to make informed decisions.
For MetaTrader5
symbol = "EURUSD"
rates = mt5.copy_rates_from(symbol, mt5.TIMEFRAME_M1, mt5.datetime(), 1000)
For Binance
ticker = client.get_symbol_ticker(symbol="BTCUSDT")
The ultimate goal of your bot is to trade. With the API integration, you can place buy or sell orders programmatically.
For MetaTrader5
order = mt5.order_send(
symbol=symbol,
action=mt5.ORDER_BUY,
volume=1,
price=mt5.symbol_info_tick(symbol).ask,
deviation=20,
type_time=mt5.ORDER_TIME_GTC,
type_filling=mt5.ORDER_FILLING_IOC,
)
For Binance
order = client.create_order(
symbol='BTCUSDT',
side=Client.SIDE_BUY,
type=Client.ORDER_TYPE_LIMIT,
timeInForce=Client.TIME_IN_FORCE_GTC,
quantity=1,
price='10000'
)
Always be prepared for the unexpected. Ensure your bot can handle errors gracefully, be it a failed trade execution or a lost connection.
try:
# Your trading logic here
except Exception as e:
print(f"Error encountered: {e}")
Volatility is the lifeblood of the RORO strategy. It’s the measure of price fluctuations in an asset over a specific period. By gauging volatility, traders can determine the risk environment and adjust their strategies accordingly. A trading bot that can accurately measure and respond to volatility has a significant edge in the market.
Before diving into the technicalities, it’s essential to grasp what volatility signifies:
For our RORO bot, we’ll focus on historical volatility, specifically using the Average True Range (ATR) as our indicator.
Developed by J. Welles Wilder, ATR measures market volatility. It’s calculated by taking the average of the true range over a specified period. The true range is the greatest of:
Using Python, and specifically the Pandas library, the ATR can be computed as follows:
import pandas as pd
def calculate_atr(data, period=14):
data['HL'] = data['High'] - data['Low']
data['HC'] = abs(data['High'] - data['Close'].shift())
data['LC'] = abs(data['Low'] - data['Close'].shift())
data['TR'] = data[['HL', 'HC', 'LC']].max(axis=1)
data['ATR'] = data['TR'].rolling(window=period).mean()
return data['ATR']
A higher ATR indicates higher volatility, while a lower ATR suggests lower volatility. For the RORO strategy:
With the ATR calculated, it can be integrated into the bot’s decision-making process. For instance, if the ATR crosses a certain threshold, the bot might switch from a ‘Risk On’ to a ‘Risk Off’ strategy.
def decide_trade(data, atr_threshold):
current_atr = data['ATR'].iloc[-1]
if current_atr > atr_threshold:
return "Risk Off"
else:
return "Risk On"
The ATR’s sensitivity can be adjusted by changing the period over which it’s calculated. A shorter period will make the ATR more responsive to recent price changes, while a longer period will smooth out the ATR, making it less sensitive to short-term fluctuations.
The trading logic is the brain of your bot. It’s where decisions are made based on the data and indicators at hand. For a RORO strategy, the primary decision pivot is the volatility of the market. However, merely having data isn’t enough; it’s the interpretation and actionable insights derived from this data that drive successful trades.
Before coding, outline the criteria that will influence your bot’s decisions. For a RORO bot, these might include:
This function will use the ATR (or other volatility indicators) to decide the trading action.
def trade_logic(data, atr_threshold):
current_atr = data['ATR'].iloc[-1]
if current_atr > atr_threshold:
return "SELL"
elif current_atr < atr_threshold:
return "BUY"
else:
return "HOLD"
Depending on the decision made, allocate funds to the appropriate assets. For instance, during ‘Risk On’ scenarios, you might want to invest more in equities, while during ‘Risk Off’ scenarios, bonds might be more appealing.
def allocate_funds(decision, portfolio_value):
if decision == "BUY":
return portfolio_value * 0.8 # 80% allocation to riskier assets
elif decision == "SELL":
return portfolio_value * 0.2 # 20% allocation to riskier assets
else:
return portfolio_value * 0.5 # Neutral allocation
To further refine your trading logic, incorporate stop-loss and take-profit mechanisms. These automatically sell assets when they hit a certain loss or profit threshold, adding an extra layer of risk management.
def set_stop_loss(current_price, percentage):
return current_price - (current_price * percentage/100)
def set_take_profit(current_price, percentage):
return current_price + (current_price * percentage/100)
Markets evolve, and what worked yesterday might not work today. Continuously monitor your bot’s performance and adjust the trading logic as needed. This might involve tweaking the ATR threshold or adjusting asset allocation ratios.
In the world of trading, unexpected events are the norm. Ensure your trading logic can handle exceptions, be it a sudden market crash or an API error.
try:
# Your trading logic here
except Exception as e:
print(f"Error encountered: {e}")
# Maybe send an alert or notification
Risk management is the safety net of your trading strategy. It ensures that even during unfavorable market conditions, your losses are contained, and your capital is preserved. Without proper risk management, even the most sophisticated trading logic can lead to significant losses.
Determine the maximum percentage of your portfolio you’re willing to risk in a single trade. This could be a fixed percentage or a dynamic value based on market conditions.
These are pre-determined price levels at which your bot will automatically sell an asset, either to prevent further losses or to lock in profits.
def set_stop_loss(current_price, percentage):
return current_price - (current_price * percentage/100)
def set_take_profit(current_price, percentage):
return current_price + (current_price * percentage/100)
Avoid putting all your eggs in one basket. Diversify your investments across various assets to spread and mitigate risks.
Continuously assess the risk parameters and adjust them based on the bot’s performance and market conditions.
Backtesting is the process of testing your bot’s trading logic against historical market data. It provides insights into how the bot would have performed in the past, which can be indicative (though not guaranteed) of future performance.
Source comprehensive data for the assets you’re interested in. This data should span various market conditions to test the bot’s robustness.
Run your bot’s trading logic against this data, simulating buy and sell actions based on the strategy.
Evaluate the bot’s performance. Key metrics to consider include:
Based on backtesting results, tweak your trading logic, risk parameters, and other elements of your strategy.
The financial market is a living entity, constantly evolving. To ensure sustained profitability, it’s crucial to monitor your bot’s performance in real-time and make necessary optimizations.
Set up dashboards or alerts to keep track of your bot’s trades, wins, losses, and other vital metrics.
At regular intervals, review the bot’s performance. This could be monthly, quarterly, or annually.
Markets can shift due to various factors, from geopolitical events to technological advancements. Be ready to adjust your strategy in response to these changes.
import pandas as pd
import MetaTrader5 as mt5
# Assuming you also want to integrate with Binance
# from binance.client import Client
# Initialize MetaTrader5
if not mt5.initialize():
print("Initialization failed")
mt5.shutdown()
# For Binance (if you decide to use it)
# API_KEY = 'YOUR_API_KEY'
# API_SECRET = 'YOUR_API_SECRET'
# client = Client(API_KEY, API_SECRET)
def calculate_atr(data, period=14):
data['HL'] = data['High'] - data['Low']
data['HC'] = abs(data['High'] - data['Close'].shift())
data['LC'] = abs(data['Low'] - data['Close'].shift())
data['TR'] = data[['HL', 'HC', 'LC']].max(axis=1)
data['ATR'] = data['TR'].rolling(window=period).mean()
return data['ATR']
def trade_logic(data, atr_threshold):
current_atr = data['ATR'].iloc[-1]
if current_atr > atr_threshold:
return "SELL"
elif current_atr < atr_threshold:
return "BUY"
else:
return "HOLD"
def allocate_funds(decision, portfolio_value):
if decision == "BUY":
return portfolio_value * 0.8 # 80% allocation to riskier assets
elif decision == "SELL":
return portfolio_value * 0.2 # 20% allocation to riskier assets
else:
return portfolio_value * 0.5 # Neutral allocation
def set_stop_loss(current_price, percentage):
return current_price - (current_price * percentage/100)
def set_take_profit(current_price, percentage):
return current_price + (current_price * percentage/100)
# Sample usage
data = pd.DataFrame({
'High': [1.15, 1.18, 1.20, 1.14],
'Low': [1.10, 1.14, 1.16, 1.09],
'Close': [1.14, 1.17, 1.18, 1.10]
})
atr_values = calculate_atr(data)
decision = trade_logic(data, 0.03) # Assuming 0.03 as a sample ATR threshold
allocation = allocate_funds(decision, 1000) # Assuming a portfolio value of 1000
print(f"ATR Values: {atr_values}")
print(f"Trading Decision: {decision}")
print(f"Funds Allocation: {allocation}")
# Fetching real-time market data from MetaTrader5
def fetch_market_data(symbol, timeframe=mt5.TIMEFRAME_M1, count=1000):
rates = mt5.copy_rates_from(symbol, timeframe, mt5.datetime(), count)
df = pd.DataFrame(rates)
df['time'] = pd.to_datetime(df['time'], unit='s')
return df[['time', 'open', 'high', 'low', 'close']]
# Execute trades on MetaTrader5
def execute_trade(symbol, action, volume=1, deviation=20):
if action == "BUY":
order_type = mt5.ORDER_BUY
price = mt5.symbol_info_tick(symbol).ask
elif action == "SELL":
order_type = mt5.ORDER_SELL
price = mt5.symbol_info_tick(symbol).bid
else:
return None
order = mt5.order_send(
symbol=symbol,
action=order_type,
volume=volume,
price=price,
deviation=deviation,
type_time=mt5.ORDER_TIME_GTC,
type_filling=mt5.ORDER_FILLING_IOC,
)
return order
# Main function to run the bot
def run_bot(symbol, atr_threshold, portfolio_value):
data = fetch_market_data(symbol)
data['ATR'] = calculate_atr(data)
decision = trade_logic(data, atr_threshold)
allocation = allocate_funds(decision, portfolio_value)
# Execute the trade based on the decision
order_result = execute_trade(symbol, decision)
if order_result and order_result.retcode == mt5.TRADE_RETCODE_DONE:
print(f"Trade {decision} executed successfully for {symbol}")
else:
print(f"Trade {decision} failed for {symbol}. Reason: {order_result.comment if order_result else 'Unknown'}")
return {
"ATR Values": data['ATR'].tolist(),
"Trading Decision": decision,
"Funds Allocation": allocation
}
# Sample usage
result = run_bot("EURUSD", 0.03, 1000)
print(result)