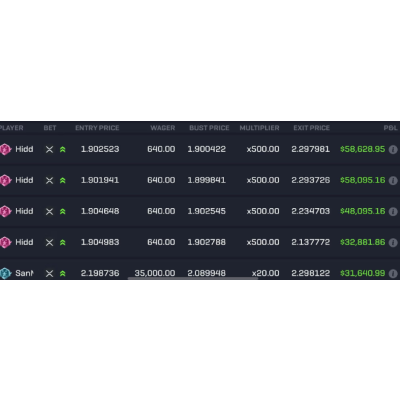
Strategies come and go, but a few remain timeless. One such strategy is the Mean Reversion. At its core, it’s based on the principle that asset prices and historical returns eventually revert to their long-term mean or average level. By leveraging this principle, traders can capitalize on price deviations. In today’s digital age, automating this strategy through a bot can provide a competitive edge. Let’s dive into how to create a Mean Reversion Bot.
The foundation of any successful trading strategy lies in a deep understanding of its core principles. For the Mean Reversion Bot, it’s essential to grasp the idea that prices, over time, tend to revert to their historical average. This reversion can be due to various factors, such as market corrections, external news, or simply the natural ebb and flow of trading dynamics.
Picture a pendulum. After swinging to one side, it inevitably returns to its resting position before swinging again. This is analogous to asset prices in the financial markets. When prices swing too far in one direction, they often revert, providing traders with potential opportunities. By understanding this principle, you’re not predicting the future but rather recognizing a pattern that has consistently played out in the past.
The mean reversion principle is rooted in the belief that while short-term price movements can be influenced by a myriad of unpredictable factors, long-term movements tend to be more predictable as they gravitate towards a mean or average. This doesn’t mean that prices will always revert quickly, but over a longer timeframe, the odds increase. Recognizing and capitalizing on these odds is where the Mean Reversion Bot comes into play.
In the world of trading, the saying “Garbage in, garbage out” holds. The quality and accuracy of the data you feed into your bot will directly impact its performance. For a Mean Reversion Bot, historical price data is the cornerstone. This data allows the bot to calculate averages, identify deviations, and make informed trading decisions.
Consider a chef preparing a gourmet dish. The outcome is heavily reliant on the quality of ingredients used. Similarly, for a trading bot, high-quality data is the primary ingredient. Without it, even the most sophisticated algorithms can falter.
Data collection isn’t just about gathering vast amounts of information but ensuring its relevance and accuracy. For mean reversion, it’s crucial to have data that spans various market conditions, from bull runs to bearish downturns. This comprehensive dataset allows the bot to learn and adapt to different scenarios. Furthermore, data management involves cleaning the data (removing anomalies or outliers), normalizing it (ensuring consistency), and storing it efficiently for quick retrieval. Modern tools and databases, such as pandas in Python or SQL databases, can aid in these tasks.
import pandas as pd
# Load data from a reliable source
data = pd.read_csv('historical_prices.csv')
# Clean and preprocess data
data.dropna(inplace=True)
data['Date'] = pd.to_datetime(data['Date'])
data.set_index('Date', inplace=True)
The moving average is a pivotal component in the mean reversion strategy. It provides a smoothed representation of an asset’s price over a specific period, helping to filter out the noise of daily price fluctuations. By comparing the current price to this average, traders can identify potential overbought or oversold conditions, signaling potential reversion opportunities.
Imagine you’re on a hiking trail, and you want to gauge your overall altitude trend. While individual steps might take you up or down, the trail’s average altitude gives you a clearer picture of your general elevation. Similarly, in trading, the moving average offers a clearer view of an asset’s general price direction, helping traders spot deviations from the norm.
There are various types of moving averages, such as the Simple Moving Average (SMA) and the Exponential Moving Average (EMA). The SMA calculates the average of price data over a set number of periods, while the EMA gives more weight to recent prices, making it more responsive to recent price changes. The choice between them depends on the trader’s preference and the specific strategy employed. For a basic mean reversion bot, the SMA is a good starting point due to its simplicity.
The window or period of the moving average is also crucial. A shorter window (e.g., 20 days) will be more sensitive to price changes, while a longer window (e.g., 200 days) will be smoother and less reactive. The choice of window should align with the trader’s horizon and the frequency of trading signals desired.
# Calculate the 50-day Simple Moving Average
data['50_SMA'] = data['Price'].rolling(window=50).mean()
# For those interested in the Exponential Moving Average
data['50_EMA'] = data['Price'].ewm(span=50, adjust=False).mean()
While the moving average provides a reference point, it’s the deviation from this average that signals trading opportunities. However, not all deviations are equal. Minor fluctuations might be mere noise, while significant deviations could indicate genuine overbought or oversold conditions. Setting a clear threshold for deviation ensures that the bot reacts only to meaningful price movements, reducing the risk of false signals.
Think of the threshold as a safety buffer. If you’re waiting for a bus, you wouldn’t run after it the moment it’s a foot away from the stop. You’d wait for a clear indication that it’s leaving. Similarly, in trading, the threshold ensures you’re not reacting to every minor price movement but waiting for a clear sign that the asset is deviating from its norm.
The threshold can be set in various ways. A common approach is to use standard deviations, which measure the amount of variation or dispersion from the average. A higher standard deviation indicates higher volatility and vice versa. By setting the threshold as a multiple of the standard deviation, traders can adjust their sensitivity to price movements. For instance, a 2-standard deviation threshold would capture roughly 95% of price movements, making deviations beyond this point statistically significant.
Another approach is to set a fixed percentage from the moving average. For instance, a 2% threshold would trigger a signal if the price moves 2% above or below the moving average. The choice between these methods (or a combination of both) depends on the asset being traded, market conditions, and the trader’s risk tolerance.
# Using standard deviation as a threshold
std_dev = data['Price'].rolling(window=50).std()
data['Upper_Bound'] = data['50_SMA'] + (2 * std_dev)
data['Lower_Bound'] = data['50_SMA'] - (2 * std_dev)
# Alternatively, using a fixed percentage
percentage_threshold = 0.02
data['Upper_Bound_Percent'] = data['50_SMA'] * (1 + percentage_threshold)
data['Lower_Bound_Percent'] = data['50_SMA'] * (1 - percentage_threshold)
The trading logic is the heart of the Mean Reversion Bot. It’s where decisions are made based on the data and the parameters set. While the previous steps laid the groundwork by providing the necessary data points, the trading logic translates these into actionable buy, sell, or hold signals. Without a clear and effective trading logic, the bot would be directionless, unable to capitalize on the insights derived from the data.
Consider a car’s engine. While the vehicle might have the best tires, brakes, and fuel, it’s the engine that propels it forward. Similarly, the trading logic is what drives the bot, propelling it to make decisions that can lead to profitable trades. A well-defined logic ensures that the bot operates efficiently, maximizing potential returns while minimizing risks.
The trading logic for a Mean Reversion Bot typically involves comparing the current price to the bounds set by the threshold. If the price breaches the upper bound, it might be considered overbought, signaling a potential sell opportunity. Conversely, if it falls below the lower bound, it might be deemed oversold, indicating a potential buy opportunity. However, it’s essential to incorporate additional checks and balances, such as ensuring the bot doesn’t trade too frequently or considering other technical indicators to confirm signals.
Additionally, the logic can be enhanced by incorporating stop-losses, take-profit points, and other risk management techniques to protect the portfolio and lock in profits.
def trade_logic(row):
if row['Price'] > row['Upper_Bound']:
return "Sell"
elif row['Price'] < row['Lower_Bound']:
return "Buy"
else:
return "Hold"
data['Action'] = data.apply(trade_logic, axis=1)
Before deploying any trading strategy in a live environment, it’s imperative to understand its historical performance. Backtesting provides this hindsight by simulating the bot’s trading decisions on past data. It offers insights into potential profitability, drawdowns, and the strategy’s overall risk profile. Without backtesting, traders would be venturing into the market blind, relying on hope rather than empirical evidence.
Imagine you’ve designed a new aircraft. Would you allow passengers on board without testing its flight capabilities? Similarly, backtesting is the dry run for your trading bot, ensuring it can “fly” in the market conditions without crashing.
Backtesting involves running the trading logic on historical data and recording the results. It provides metrics like total returns, Sharpe ratio, maximum drawdown, and trade win percentage. These metrics help in assessing the strategy’s robustness and its ability to weather different market conditions.
However, it’s crucial to be wary of overfitting, where the strategy performs exceptionally well on historical data but falters in real-time trading. This can happen if the strategy is too complex or tailored too closely to past data anomalies.
# Simple backtesting logic
initial_balance = 100000
balance = initial_balance
for index, row in data.iterrows():
if row['Action'] == "Buy" and balance >= row['Price']:
balance -= row['Price']
elif row['Action'] == "Sell" and balance >= 0:
balance += row['Price']
final_balance = balance
performance = (final_balance - initial_balance) / initial_balance * 100
The financial markets are ever-evolving, influenced by a myriad of factors ranging from geopolitical events to technological advancements. A strategy that works today might not be as effective tomorrow. Continuous monitoring ensures that the bot is performing as expected, while optimization tweaks the strategy to adapt to changing market conditions.
Consider a professional athlete. Even after reaching the pinnacle of their sport, they continuously train and adapt to maintain their edge. Similarly, a trading bot requires ongoing refinement to stay competitive in the dynamic world of finance.
Monitoring involves regularly checking the bot’s performance metrics, ensuring they align with the results from backtesting. Any significant divergence might indicate issues, such as data feed problems or changes in market behavior.
Optimization, on the other hand, is about fine-tuning. This could involve adjusting the threshold for deviation, incorporating additional technical indicators, or even redefining the entire trading logic. Advanced techniques like machine learning can also be employed to optimize parameters automatically.
It’s essential to strike a balance between reactivity and stability. While it’s crucial to adapt to the market, frequent changes can lead to overfitting and increased transaction costs.
# Sample optimization logic using a grid search approach
best_performance = -float('inf')
best_threshold = None
for threshold in range(1, 5):
# ... run backtesting with the current threshold ...
if current_performance > best_performance:
best_performance = current_performance
best_threshold = threshold
import pandas as pd
# 2. Data Collection and Management
# Load data from a reliable source
data = pd.read_csv('historical_prices.csv')
# Clean and preprocess data
data.dropna(inplace=True)
data['Date'] = pd.to_datetime(data['Date'])
data.set_index('Date', inplace=True)
# 3. Calculating the Moving Average
# Calculate the 50-day Simple Moving Average
data['50_SMA'] = data['Price'].rolling(window=50).mean()
# 4. Setting the Threshold for Deviation
# Using standard deviation as a threshold
std_dev = data['Price'].rolling(window=50).std()
data['Upper_Bound'] = data['50_SMA'] + (2 * std_dev)
data['Lower_Bound'] = data['50_SMA'] - (2 * std_dev)
# 5. Implementing the Trading Logic
def trade_logic(row):
if row['Price'] > row['Upper_Bound']:
return "Sell"
elif row['Price'] < row['Lower_Bound']:
return "Buy"
else:
return "Hold"
data['Action'] = data.apply(trade_logic, axis=1)
# 6. Backtesting
initial_balance = 100000
balance = initial_balance
for index, row in data.iterrows():
if row['Action'] == "Buy" and balance >= row['Price']:
balance -= row['Price']
elif row['Action'] == "Sell" and balance >= 0:
balance += row['Price']
final_balance = balance
performance = (final_balance - initial_balance) / initial_balance * 100
# 7. Continuous Monitoring and Optimization (Sample Optimization Logic)
best_performance = -float('inf')
best_threshold = None
for threshold in range(1, 5):
# ... run backtesting with the current threshold ...
if current_performance > best_performance: # Note: 'current_performance' needs to be defined based on the backtesting results for each threshold
best_performance = current_performance
best_threshold = threshold
print(f"Optimal Threshold: {best_threshold}")
print(f"Final Performance: {performance}%")
Note: This code is a simplified version of a Mean Reversion Bot and serves as a starting point. In a real-world scenario, additional functionalities, error handling, and optimizations would be necessary. Ensure you have the required libraries installed and the ‘historical_prices.csv’ file available for this code to run successfully.