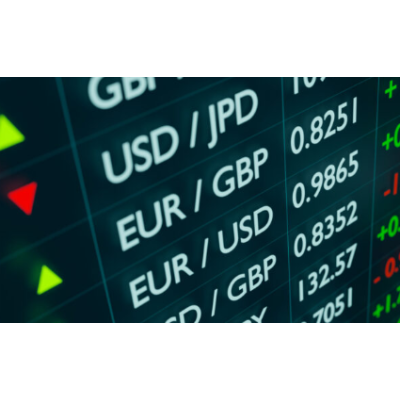
First things first, let’s address the elephant in the room: there are countless trading bots available online, many with impressive track records and a long list of satisfied customers.
So, you might be wondering, why in the world would you want to build your own?
Well, apart from the obvious thrill and satisfaction of creating something from scratch, building your own trading bot is a fun and hands-on way to understand the ins and outs of the cryptocurrency world. Plus, it allows you to experiment with Node.js and Binance API, two powerful tools you’ll be using a lot if you delve deeper into the crypto universe. However, it’s important to note that the bot we’re building here is for educational purposes, and may not necessarily help you make a fortune overnight.
Before we start, make sure Node.js is installed on your machine. You can download it from the official Node.js website. Post-installation, you can check the successful setup with the following commands:
node --version
npm --version
These will display the installed versions of Node.js and npm, ensuring that you're good to go.
Now, let's create a new Node.js project. Here's how you can do it:
mkdir my-trading-bot
cd my-trading-bot
npm init -y
This creates a new directory called my-trading-bot
, navigates into it, and initiates a new Node.js project.
Next, we'll need the official Binance API package. Install it with the following command:
npm install binance-api-node
Now, it's time to set up your Binance API. First, you'll need to create an account on Binance if you don't already have one. Then, navigate to the API section in the user dashboard and create a new API Key. Note the API Key and Secret - we'll need them soon.
When you generate your API keys in Binance, make sure to enable the correct permissions. For this bot, you need at least Enable Reading
and Enable Spot & Margin Trading
permissions.
In order to execute trades on Binance, you will need to have funds available in your account. This could be in the form of Bitcoin or other cryptocurrencies, or you could deposit fiat currency (like USD, EUR, etc.) from a registered bank account or credit card.
With the environment all set, it's time to start writing our bot. Create a new bot.js
file in your project directory:
touch bot.js
Open bot.js
in your favorite text editor, and start off by importing the packages we installed earlier:
const Binance = require('binance-api-node').default;
// Authenticate with your API keys
const client = Binance({
apiKey: 'your-api-key',
apiSecret: 'your-api-secret',
});
Now you're ready to start programming your bot. The strategies and trading logic can be as simple or as complex as you wish. For this tutorial, let's keep it simple: our bot will place a market order to buy 0.001 BTC when it reaches a certain price.
async function run() {
const price = await client.prices();
if (parseFloat(price['BTCUSDT']) < 31000) {
console.log('Buying BTC at low price!');
await client.order({
symbol: 'BTCUSDT',
side: 'BUY',
quantity: 0.001,
type: 'MARKET'
});
}
}
run();
That's it! You've just written your first trading bot. It's a basic example, but it illustrates the principles of using Node.js and the Binance API to automate trading decisions.
Important Notice
Before we wrap up, it’s crucial to understand that when you run this script, you will be executing real transactions with your actual funds on Binance. The bot we’ve written in this tutorial will attempt to purchase Bitcoin with your real money if the Bitcoin price falls below the limit we’ve set.
Remember, every time you run the bot, it may potentially place an order, which could lead to real financial loss if not monitored and managed carefully. Always double-check your code and test it under controlled circumstances before letting it handle your trades.
To test your bot, you can run node bot.js
in your terminal.
Do remember that this is a real transaction - your bot will attempt to buy BTC if the price is below $31,000.
Given that today’s current Bitcoin price is at $30,700, which is less than our set limit of $31,000, running the bot now would trigger a purchase. It will immediately buy Bitcoin according to the parameters we’ve set.
Let’s have a look at the results. Here’s a screenshot of my Binance wallet, now proudly displaying an additional 0.001 BTC — the fruits of our bot’s first transaction.
As you can see, our simple Node.js bot is not just a concept; it can interact with the real-world markets and execute live trades!
Before concluding this tutorial, I’d like to emphasize that while creating your own trading bot can be an exciting and educational experience, it also comes with its own set of risks and responsibilities. Here are some key points to bear in mind:
Creating a trading bot can be a rewarding project that not only educates you on the practical applications of Node.js and APIs, but also provides insights into the world of cryptocurrency trading. It’s an adventure, full of potential, just waiting for you to take the first step. Remember to have fun and stay safe.