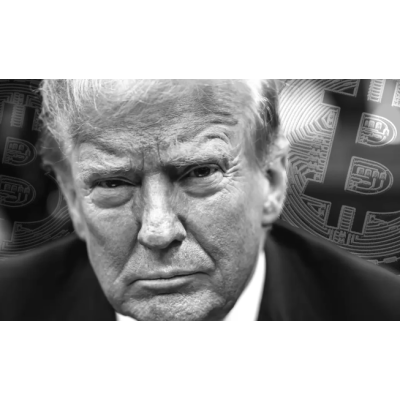
Tired of buying your cryptos too high and then selling too low? It doesn’t have to be that way! Here is a fun project for building your own trading bot. This article uses Python as it is the easiest programming language to learn (my personal opinion).
I will show you how to create a trading bot in Python that will automatically buy and sell crypto currencies via the Coinbase Cloud API based on certain trading indicators. From a technical perspective, the trading bot will be deployed on Google Cloud Platform (GCP). It uses Google Firestore to keep track of all initiated orders. The bot runs as a scheduled cloud function. Here are the things you will learn in this article:
This article focuses on the conceptual setup with a strong focus on the overall mechanics. The full working code including a step-by-step deployment instruction is referenced at the end of the article. Feel free to play around with the code and deploy it in your own Google GCP project.
The trading strategy of the bot suggested in this article is pretty straightforward but offers enough on-ramps so that you can build your own algorithm on top of it. The bot will run every five minutes to check if the following three conditions are true:
If all three conditions are met (meaning there is a good dip in the price with a positive market signal), the bot will trigger a market buy trade. Once a market trade is executed, the bot will automatically create a stop limit sell order with the goal to achieve a certain profit margin. Of course, the algorithm relies on rising market prices in the long term.
First, you need your own account with Google Cloud Platform and create a dedicated project for this article. Sign-up with GCP should be fairly easy and you probably want to take a basic tour through their platform to better understand the components we will use. It is also recommended to take their Cloud Leader certification if you have some time. We will use the following GCP features for this article: Cloud Functions, IAM, Security, Cloud Scheduler, Firestore. You also need to install the gcloud CLI on your local machine to conveniently manage your Google resources.
Second, you must have an account with Coinbase. In Coinbase, you must create your own API key and secret under Settings/API. Coinbase delays the activation of your API key and secret by 48 hours as of writing this article. Needless to say, that you also need to transfer some money to Coinbase so that the trading bot has the resources to trade cryptos.
On your local machine, you need a project folder. All Python code is in one single main.py file. Your external pip libraries are listed in requirements.txt. Environment variables are kept in file .env (only needed for local testing) and an .env.yaml (uploaded to GCP). The gcloud suite also comes with a Flask server that you can run to execute and debug your code locally.
The architecture also shows the two external APIs that we will leverage: Coinbase Cloud and Trading View.
On the GCP side, we have the investment bot cloud function as the central element. On top, we have the cloud scheduler. The third key component is the Firestore document database.
In GCP, we use the following features:
Here is the main Google Cloud function which calls two custom functions in line two and three. Both functions have a Pandas dataframe as an output. A Cloud Function cannot return a Pandas dataframe. Therefore, in line four, the dataframe is concatenated and outputted in JSON format and it is returned together with an HTTP 200 OK status:
The (simplified) sequence diagram of our crypto trading bot is as following:
The first function make_investment_decision() loads the necessary environment variables in lines 5 to 9 like the quote currency (e.g. USD or EUR) and base currencies (e.g. BTC, LTC, ETH) that you would like to use. In lines 10 to 14, the historic data, the 24 hour stats, server time and additional trading recommendations are requested via the Coinbase and Trading View APIs.
Next, the algorithm iterates through all crypto currencies you would like to trade (stored in environment variable MY_CRYPTO_CURRENCIES). Between line 17 and 26, the necessary data for decision making is prepared. The investment decision itself including a potential market order in Coinbase and the update to Firestore is done in lines 27 to 30.
The next function place_sell_orders() initiates a sell order for each purchase order from the previous step. To achieve this, it iterates through all Firestore documents that do not yet have a sell order id (line 6 to 8). For each relevant document (one document is equivalent to one market buy order), missing order data is requested from Coinbase and Firestore is updated (line 9 to 33). Next, a sales order is created in Coinbase (line 34 to 41). At last, Firestore is again updated with the details of the sales order.
In line 48, the list of sell orders is returned as a Pandas dataframe. Please take a look at the full code on my Github.
Before we can test the investment-bot function locally and deploy it to the cloud, a few initial steps must be taken on GCP:
You can test the code locally via the built-in Flask server that comes with the functions-framework of the gcloud CLI. Run this command from the folder where the main.py file resides:
functions-framework --target=investment-bot --debug
When you run the command, it will show you the local IP address under which your cloud function is available. You just need to open it in a browser and you should see an output similar to this one:
In case you run into errors, check the output of the terminal.
To deploy the investment bot to GCP, you must run the following command from the local folder containing the main.py file:
gcloud functions deploy investment-bot --runtime python37 --trigger-http --env-vars-file .env.yaml
If all goes well, you will see investment-bot as a new function within the Cloud Function section in GCP:
Edit the function to add the Service Account that you have created above. Assign both secrets from the previous step. The secrets need to exactly match to the environment variables used within the Python code (ignore the Sendgrid secret since it is not used in the simplified trading bot example):
You have the possibility to test the execution of the function from inside the function screen. If something goes wrong, check the log output. Typical errors that I have made in the past:
Once your function executes successfully on GCP, you need to create a Cloud Scheduler job so that the function is executed on a regular basis. Go to the Cloud Scheduler section and create a new scheduler job. The only tricky part is to find out the exact unix-cron format for your purpose, here is the that executes every five minutes:
*/5 * * * *
Other than this, make sure that you again assign the same Service Account to the job and that you copy and paste the exact URL that represents your Cloud Function target. Select OICD as an authorization option. The job should look similar to this one:
That’s it! You can wait for five minutes and you should see the status of your scheduler job change to Success. This means that you can now relax and the investment bot will take care of your crypto trading all by itself.