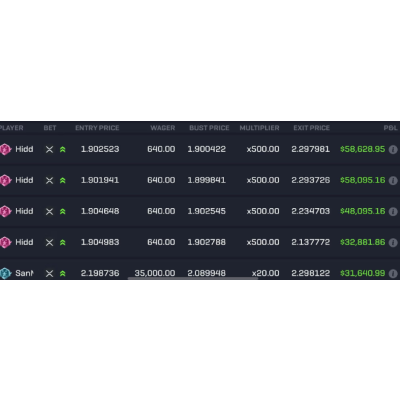
Welcome back to my series on building your own trading bot using Binance.
Crypto bot trading can be a lot of fun. There can be a lot of joy found in analyzing data, identifying trends, and then making (hopefully lots) of money from your decisions. In the stock market world, many people have stated that the discipline of quantitative analysis can make you a better trader overall.
Whatever your motivation, I hope you enjoy this series.
I could never have anticipated how popular my series and articles on algorithmic trading would become.
After a listening to my readers/viewers feedback, I realized that many people were spending huge amounts of time trying to solve installation/configuration problems — rather than experiencing the joy of algorithmic trading.
I want to change this narrative — and in so doing, open up algorithmic trading for everyone.
To do this, I’ve recently launched Tradeoxy: Trading For Everyone.
If you’re reading this, Tradeoxy will simplify 90% of this series, using a series of powerful API’s and easy to use tooling.
Currently it’s in the early-access / building stage and I’d be incredibly grateful if you’d join us on this adventure. Your feedback will help us shape a better product.
Join the early access program (for free) here.
You can also view our launch video, and follow our journey on LinkedIn, Twitter, Instagram, YouTube.
In this series, I show you how to use the REST API of Binance, the world’s largest centralized crypto trading platform, to build a crypto trading bot. Together, we build an example trading bot that identifies fast-rising crypto assets, buying them low and selling them high. By the end of the series, you’ll have everything you need to build your own trading bot. If you’d like further assistance, contact me here.
All activity is demonstrated in Python. Each episode contains working code samples, with the full code accessible on GitHub here.
Note: all code is for example use only and I make no guarantees about the strategy. Do your own research and use it at your own risk 😊.
When I first wrote this series, I could never have imagined the incredible response I received.
After the requests from many readers, I’ve since launched a YouTube Channel and website to help take this content even further. Why not drop in and say hi ❤
By the end of this episode, you’ll have built your very own trading strategy and to actively analyze market data.
Before diving into strategy development, I want to make a quick note about the strategy. The strategy I’m demonstrating should only be used at your own risk — after you do your own research. I cannot and do not make any statements about its viability.
If you do choose to use it, I only ask that you give me a quick shout-out on LinkedIn ❤
The strategy I’ll be demonstrating is designed to take advantage of momentum-based trading events. Here are the rules:
Here’s what this would look like visually:
To simplify code development, I’ll also implement the following restrictions:
Note. If you’re someone who tracks crypto pump and dump events, this strategy could be easily modified to identify these events. You could then filter these out from your trading.
Strategy implementation starts with correctly formatting data.
I’ll start by updating the function get_candlestick_data
from Episode 1 (episode list at the bottom of the article). Readers who have seen my previous series How to Build a MetaTrader 5 Python Trading Bot will recognize the data format. Without giving too much away, I’ve standardized the format in preparation for a future series 😉
The Binance API documentation for Kline/Candlestick Data explains how to interpret the results from the queries. Expressed in code, it looks like this:
If you’re following on from Episode 1, press Play on your IDE and you should see some nicely formatted results come back 😊
Next, I’ll convert this data into a Pandas DataFrame. To do this, create a new Python file called strategy.py
in your project. Add the below code to this file, noting that I’ve added a column RedOrGreen
to the original data to identify if it’s ‘Red’ (trending down) or ‘Green’ (trending up):
So far so good! Our Binance trading bot is coming along well!
The strategy provides a series of rules which can be used to identify a buy
. Here they are in normal human speak:
To implement this signal, a method is needed to analyze a given symbol. This can be done by passing each symbol into a function called determine_buy_event
. Here’s the code, placed in strategy.py
:
Note. Astute programmers will note the use of hard-coded functions for determining buy events. This could certainly be abstracted into a simple for loop. I’ve done it this way to emphasize the calculation.
Next, a method is needed to generate a list of all the signals to be analyzed. To do this, extract all the symbols with a specified base asset. Do this by adding the function query_quote_asset_list
in binance_interaction.py
:
At the time of writing, this returned 365 assets (tokens).
It’s time to analyze the symbols!
This is done through a series of logical steps:
True
or False
value for each symbolNote. Binance has strict API Request limits, explained here.
Binance has strict request limits for its API. They are certainly generous but need to be respected. To see the latest updates on the limit, check this webpage out. At the time of writing, this is 1200 weighted requests per minute (reasonably generous).
To ensure that you stay below the request limit, I’ve implemented a time.sleep
of 1 second per iteration. This doesn’t impact the performance of the algorithm, but stops your API Key from getting banned ❤
Here’s the initial code to analyze your symbols:
I’ll return to this function in a future episode.
If you’d like to play with what you’ve developed so far, update your main as below:
Make sure you’ve imported the functions we’ve developed import binance_interaction
and import strategy
then press Play on your IDE.
Here’s what mine looked like with a 10% setting: