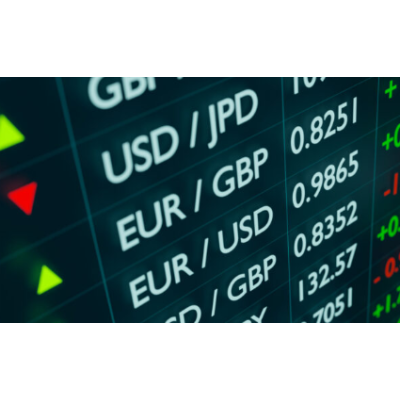
Ever wanted to build an automated trading bot? Got a strategy in mind? Got some skills in Python?
My new series “How to Build a Meta Trader 5 Python Trading Bot” was written just for you.
About the Series
The series shows you how to build your very own Python Trading Bot for Meta Trader 5 using the MetaTrader module for Python.
The series assumes that you have a working knowledge of Python scripting, know how to use an IDE and understand the basics of trading (stop loss, take profit, and so on). All code is used at your own discretion and risk, including developing your own strategy 😊
By the end of this episode, your project will be set up and MT5 will be getting started by Python.
MetaTrader5 (MT5) is one of the most widely used retail trading platforms for Foreign Exchange (Forex), Stocks, and Futures on the planet. Many large brokers (for instance IC Markets) provide it to their retail clients.
Before MT5, there was Meta Trader 4 (MT4). For our purposes, the biggest functional difference between MT4 and MT5 is the Python-based Application Programming Interface (API) implemented in MT5. This expanded the previous C++ based Meta Query Language (MQL) into the more readable and vastly more popular Python Programming Language.
For the rest of this series, you need the following:
Your project will use main.py
and three other files. Set these up now in your IDE of choice (I use Jetbrains Pycharm):
main.py
— the main function for our trading botmt5_interface.py
— our interface with MT5strategy.py
— our trading strategysettings.json
— your project settings. If you’re using a Version Control System (VCS), immediately add your settings.json to .gitignore or equivalentIt should look something like this:
For this series, all settings variables are stored in JSON format in a separate file called settings.json
. JSON is used as it is a widely used text-based format for representing structured data, especially on websites, and it is stored in a separate file to prevent the hard-coding of sensitive information into scripts.
An example of what settings.json
looks like can be found in the file example_settings.json
, linked to GitHub here. This file contains the full list of settings needed for the tutorial, with any sensitive information removed.
Let’s get settings.json
implemented.
Four variables are needed initially:
username
— the username being used for your MT5 login (demo account!)password
— the password for the MT5 loginserver
— the server your broker gives you to log in tomt5Pathway
— this is the pathway to your MT5 .exe on windowsTypically the username, password, and server variables are provided by your broker, while the mt5Pathway is where your MT5 executable is stored. Here’s what mine looked like (fake information used for username/password 😊):
{
"username": "your_username - typically an 8 digit number",
"password": "Do not share with anyone!",
"server": "ICMarkets-Demo",
"mt5Pathway": "C:/Program Files/ICMarkets - MetaTrader 5/terminal64.exe"
}
Next, you need to import these settings into the program. This will be done through main.py
Here’s the function:
In this code we:
json
and os
ImportError
if it didn’tNow, still in main.py
, add two more lines under the if __name__ == '__main__':
import_filepath = "Path/To/Your/Settings.json"
the filepath to your settingsproject_settings = get_project_settings(import_filepath)
retrieve the project settingsHere’s what your main function should look like (your filepath will probably be different):
# Main function | |
if __name__ == '__main__': | |
import_filepath = "C:/Users/james/PycharmProjects/how_to_build_a_metatrader5_trading_bot_expert_advisor/settings.json" | |
project_settings = get_project_settings(import_filepath) |
Awesome work on getting your settings imported!
The next stage is to initialize your MT5 using Python. Let’s get going!
The MetaTrader5 Python API, while extensive, can be quite fiddly. Ensuring that variables are explicitly cast to their correct type (int, float, string, etc) is always required.
Quick Note. I know for many of my advanced programming readers this is not uncommon. Especially for those who use languages like Rust, C, Go, etc. However, for those with simple Pythonic experience, this can be quite the wake-up call!
MT5 can be ‘fiddly’ to get started. In my experience, the only consistent way to ensure that it is started AND working properly is to implement two functions: initialize
and login
. Weirdly enough, there have been times when I’ve initialized but not logged in and MT5 has worked. Many tutorials only talk about the initialize
function, which can leave you stranded when it doesn’t work ❤
The function below includes both initialize
and login
. This __should__ ensure a consistent startup experience and save you many hours of troubleshooting.
Implement this function in the mt5_interface.py
file created at the start of the tutorial.
Next, in main.py
include the following:
import mt5_interface
at the top of the file__main__
, add mt5_interface.start_mt5(project_settings["username"], project_settings["password"], project_settings["server], project_settings["mt5Pathway"])
Hit Play on your IDE now and MetaTrader5 should start! Sweet!
If you go to your Journal
on MT5, you will see that your account has been authorized and synchronized:
Here’s what your main.py
should look like (a few comments added):