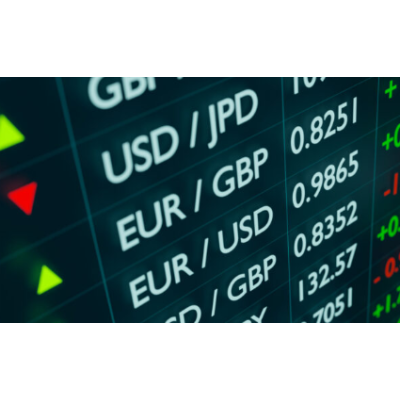
Before I start this story, I want to tell you something. I am not a financial advisor, and the trading bot we are going to build is not about making money. Algorithmic trading is a whole, and you can’t make money just by reading a few stories on Medium.
Likewise, a trading bot is not a magical thing. It won’t make you money just like that. You need to have both programming and trading skills to be able to make money this way.
So please, consider this series educational. And eventually, with a little more knowledge and work on your part, you will be able to go beyond this series and go more in-depth into trading bots to don’t lose money.
Are you learning programming? Or maybe you’re just interested in algorithmic trading? No matter, building a trading bot is an interesting project for every programmer wanting to acquire some more knowledge.
I’ll guide you through this project to explain it to you step by step. First, I’ll become with an introduction (this story). It will be very general because the purpose is only to give the key concepts. Everyone interested in building a trading bot can read it. Then I’ll share a concrete implementation of it in Python. If you’re not a Pythonista, perhaps it won’t be interesting for you.
To build a trading bot, you’ll need only two tools:
You can build a trading bot in many programming languages. In the next of this series, we’ll do it with Python. Another possibility could have been JavaScript using a Node.JS environment. And yet another possibility would have been MQL (if you know Metatrader, you know what I’m talking about).
But more generally, building a trading bot is possible with every programming language, it will just be easier or harder with some.
Then, about the server. It’s just the thing sending requests to APIs. It is what allows us to interact with the outside world, with a broker for example.
The choice depends on what you need. For testing, you can just run the code from your computer. But if you want your code to be in production and run all the time, it’s definitely not a good option.
For me, I run my trading bot on a Raspberry Pi. It’s a fun and cool option. If you want to go with it, just do it. Having a Pi is not a waste of money as it can be useful for other projects.
The other option is running code on the cloud, which is perhaps a better option. There are solutions for this like AWS or Azure. It can even be free as some cloud providers offer a free tier that should meet your needs.
You should try to abstract your bot as much as possible. I mean, you don’t want to make a Binance trading bot for example (or perhaps you want, I don’t know). You want to make a trading bot.
It means, no matter the exchange, no matter the market, no matter… the bot will work. One big error I’ve done when building my first trading bot is that I’ve built it only for Binance. When I wanted to begin Forex algorithmic trading, I had to build a new bot from scratch.
So, your bot should be the more abstract as possible. It should not depend on an exchange. It should not depend on a market. It should not depend on the infrastructure it will run on. It should not depend on [fill this text with whatever you want].
What should your bot do? Should it be able to backtest? Should it be able to place stop orders? Etc…
Thanks to the abstraction principle, it’s not a problem if your needs evolve while programming your trading bot. But as general advice in programming, it’s important to define your needs before beginning a project.
It’s a little project, so you don’t need to do a strict specification, you just need to know where you want to take your project.
An example of specifications you can do:
Then, you can do a bot actions flowchart. It’s useful because you will have an overview of what you need to develop. You will be able to distinguish some big blocks of the project through this chart.
For example:
Thanks to this flowchart (make it graphically, here I wrote it but it’s better to have a visual representation) we can distinguish some big blocks:
Thanks to our flowchart, we can begin to think about some functions our abstractions will need. It can also make us think about some more abstractions.
For example, a data storage abstraction will need some functions to write data and read data. But what if we want to write data somewhere, but read it elsewhere? We need to make a Data Writer and a Data Reader.
Our flowchart can also make us think about our trading bot loop, and more generally how it will work.
First, we need to initialize our bot. It implies a connection to the database and the read of some setup data for example. Then, we have to check every x minutes/hours/days if our conditions are met, etc…
That’s all for the main concepts. As you can see, I tried to be very general so that the non-Pythonistas people can also learn something.
If you have suggestions about what you want to learn in the continuation of this series, tell me. It can be suggestions about what I should implement in the trading bot. You can define the specifications for this project if you want so that I can teach you the things you want to learn. Just comment on what you want.
Otherwise, I will make the specifications myself, and it will probably be a live trading and backtesting bot, storing data locally.
I hope this story was useful, be sure to follow me if you don’t want to miss the continuation of this series!